Just a simple cube created with Basil.js and InDesign
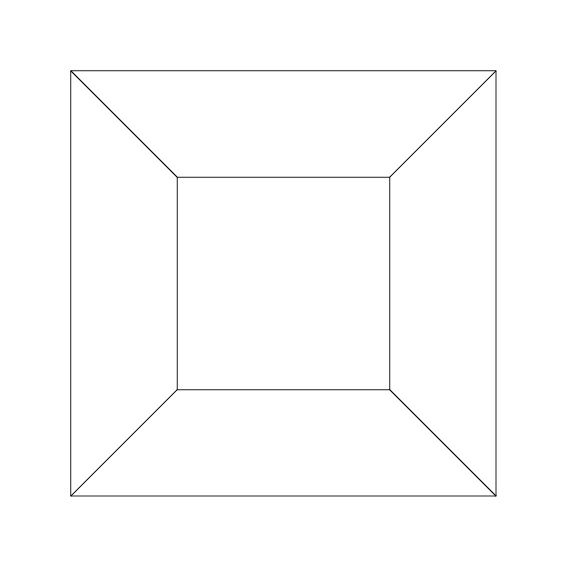
//@includepath "~/Documents/;%USERPROFILE%Documents"; // eslint-disable-line
//@include "basiljs/bundle/basil.js"; // eslint-disable-line
// based on this flash tutorial
// Scripting 3D in Flash
// by senocular
// https://www.kirupa.com/developer/actionscript/3dindex.htm
//
// TODO: expolore the tutorial even more (e.g. camera)
//
var pw = 200; // for easier handling
var ph = 200; // for easier handling
// see https://en.wikipedia.org/wiki/Focal_length
var focalLength = 300;
/**
* creates a 3D point
* @param {Number} x the x location
* @param {Number} y the y location
* @param {Number} z the z location
* @return {Object} an Object with {x:Nmber, y:Number, z:Number}
*/
var point = function(x, y, z) {
var point = {};
point.x = x;
point.y = y;
point.z = z;
return point;
};
/**
* Converts a 3D point Object to screen coordiantes
* @param {Object} pointIn3D an Object created by the point function
* @return {Object} an Object with {x:Number, y:Number}
*/
var toScreen = function(pointIn3D) {
var pointIn2D = {};
var scaleRatio = focalLength / (focalLength + pointIn3D.z);
pointIn2D.x = pointIn3D.x * scaleRatio;
pointIn2D.y = pointIn3D.y * scaleRatio;
return pointIn2D;
};
function draw() {
b.clear(b.doc()); // clear the current document
b.units(b.MM); // we want to print. use MM instead of default pixels
var doc = b.doc(); // a reference to the current document
// set some preferneces of the document for better handling
doc.documentPreferences.properties = {
pageWidth: pw,
pageHeight: ph
}; // set the page size
doc.viewPreferences.rulerOrigin = RulerOrigin.SPREAD_ORIGIN; // upper left corner
// ----------
// main code goes here
// define some 3D points
points3D = [
point(-50, -50, -100),
point(50, -50, -100),
point(50, -50, 100),
point(-50, -50, 100),
point(-50, 50, -100),
point(50, 50, -100),
point(50, 50, 100),
point(-50, 50, 100)
];
var points2D = []; // will hold the 2D points
// convert them from 3D to 2D
for (var i = 0; i < points3D.length; i++) {
p = points3D[i];
points2D[i] = toScreen(p);
}
b.pushMatrix(); // push the matrix
b.translate(b.width / 2, b.height / 2); // to the center of the screen
// draw the top plane. Could also be a polygon
b.line(points2D[0].x, points2D[0].y, points2D[1].x, points2D[1].y);
b.line(points2D[1].x, points2D[1].y, points2D[2].x, points2D[2].y);
b.line(points2D[2].x, points2D[2].y, points2D[3].x, points2D[3].y);
b.line(points2D[3].x, points2D[3].y, points2D[0].x, points2D[0].y);
// draw the bottom plane
b.line(points2D[4].x, points2D[4].y, points2D[5].x, points2D[5].y);
b.line(points2D[5].x, points2D[5].y, points2D[6].x, points2D[6].y);
b.line(points2D[6].x, points2D[6].y, points2D[7].x, points2D[7].y);
b.line(points2D[7].x, points2D[7].y, points2D[4].x, points2D[4].y);
// // connecting bottom and top planes
b.line(points2D[0].x, points2D[0].y, points2D[4].x, points2D[4].y);
b.line(points2D[1].x, points2D[1].y, points2D[5].x, points2D[5].y);
b.line(points2D[2].x, points2D[2].y, points2D[6].x, points2D[6].y);
b.line(points2D[3].x, points2D[3].y, points2D[7].x, points2D[7].y);
b.popMatrix();// reset the matrix
// ----------
var fname = File($.fileName).parent.fsName + '/' + ($.fileName.split('/')[$.fileName.split('/').length - 1]).split('.')[0] + '.indd';
// b.println(fname);
doc.save(fname, false, 'basil', true);
b.savePNG('out.png');
}
b.go();